Getting Started with AndDoneJS
This page will take you through the process of implementing AndDoneJS with step-by-step instructions on setting up and running a sample project.
Requirements
- Basic knowledge of HTML, CSS, and Javascript
- A basic code editor (Visual Studio Code)
- Access to your computer's Terminal or Powershell
- You will need Node.js installed on your system to use this sample project locally. However, for your final implementation you may use whichever server side technology your application uses.
Clone the repository
To get started, you will need to clone the sample project repository. Open a new project folder in your terminal or in Visual Studio Code. If you are using Visual Studio Code, you can open the Terminal by clicking Terminal > New Terminal in the menu bar.
The sample project repository is located at this Github link. If you prefer to clone the repository directly to your sample project, copy and paste one of the following code snippets directly into your terminal:
Using https:
git clone https://github.com/AndDone-LLC/anddone-widget.git
cd AndDone-widget-sample-payment
Using ssh:
git clone [email protected]:AndDone-LLC/anddone-widget.git
cd AndDone-widget-sample-payment
Set up your environment variables
To use the AndDoneJS embeddable widget, you will need your unique API and APP Keys. You can find your APP Key and API Key in your AndDone Agent Portal.
To avoid displaying your APP Key and API Key publicly in your code, it is recommended that you set up environment variables for them so that you can reference them at runtime. In your terminal, copy the .env.example
file to a new file named .env
.
cp .env.example node/.env
In the.env
file set the X-APP-KEY
and X-API-KEY
variables to your unique API Key and APP Key.
Important! The .env
file is to be used only for the purpose of this sample project. Never execute a production application from an environment file containing secrets.
Run the sample server
The sample server contains code that creates a payment intent request and returns the payment intent token which is required to initialize AndDoneJS.
From the sample project directory, enter the following command to install the necessary dependencies from the package.json
file.
npm i
You are now ready to start the sample project server by entering the following command.
node server.js
Once started, the sample server can accessed at http://localhost:4200
.
Configuring AndDoneJS on Your Web Page
Next, add the required code into the HTML page where you would like to embed AndDoneJS. You can refer to the index.html
file in the sample project directory to see the implementation of the steps described in this section
Include the andDone.js File
Add the following HTML snippet to the head
section of the HTML page. By adding this code into your HTML page, you are telling your HTML file to load the javascript code for the AndDoneJS widget.
<script src="https://paymentwidget.uat.anddone.com/andDone.js"></script>
Note: To be compliant, always load andDone.js from paymentwidget.anddone.com. Do not create your own bundle or install andDone.js on your hosted server.
Add supporting scripts
This sample project uses two supporting JavaScript files:
constants.js
- This file stores the amount, title, and expiresIn values that are used to generate the payment intent request. It also stores the intent token that is required to initialize AndDoneJS. You will configure these values in a later step.checkout.js
- This file contains the code that is used to generate a payment intent based on the amount, title, and expiresIn values specified inconstants.js
. When you are ready to use AndDoneJS in a production environment, you can usecheckout.js
as a code template to generate a payment intent.
Add the following HTML snippets to the head
section of the HTML page AFTER the snippet that loads andDone.js
:
<script src="./constants.js"></script>
<script src="./checkout.js"></script>
Setup configurable values
Now you need to set up your client-side configurable values found in the constants.js
file below.
Since this is a sample project, The newPaymentIntentToken value from constants.js
is set to empty (""). In order to be able to run AndDoneJS you must include checkout.js
in your HTML page AFTER loading constants.js
.
Note:checkout.js
is responsible for generating a test payment intent based on the amount, title, and expiresIn values specified inconstants.js
. When you are ready to use AndDoneJS in a production environment, thecheckout.js
file can be used as a template for your actual order information.
Create a payment container
Next you will specify where to display AndDoneJS on your web page. To do this, you will need to create a display container. You may use either a default container or a dynamic container:
Default Container
This method uses the HTML property payment-container
. AndDoneJS looks for this container by default. If it is not found, then it will look for the dynamic container as described in the next section. Add an empty placeholder div
with the property payment-container
where you would like to load the AndDoneJS form in your HTML page.
<!-- default container -->
<div payment-container ></div>
Dynamic Container
This method will make use of HTML tag IDs to find where to display AndDoneJS. Th dynamic container requires the use of a pluginOption
object which will allow you to customize AndDoneJS to match your website's theme.
Add an empty div to your checkout page with a unique id. This id will need to be passed in to the pluginOption
object when initializing AndDoneJS below. The sample code below uses mywidget
as the container id.
<!-- Dynamic container -->
<div id="mywidget" ></div> //id of the container can be of your choice
Note: It is mandatory to pass a pluginOption
object, when using a dynamic container.
The Payment Intent
As stated previously, in order to initialize the AndDoneJS instance and process a payment, you will need a payment intent token. The payment intent token is a unique key returned from AndDone as part of a payment intent request.
In this sample project, the checkout.js
file contains the JavaScript code that will generate a payment intent reqeust and return a payment intent token. When you are configuring AndDoneJS in your production environment you can use the code in checkout.js
as a template for submitting a payment intent request and generating a payment intent token.
Initializing AndDoneJS
Next, add the code to your web page to initialize the AndDoneJS widget. This code should be run only AFTER successfully receiving the PaymentIntent token.
Add the code below either in a javascript file linked to your HTML page or place it directly into your HTML page in a <script>
tag. The code should be placed in the HTML footer
section or at the end of the page body
. This is to ensure the DOM is fully loaded before the code is called.
let andDone = new AndDone(paymentIntentToken);
//OR
let andDone = new AndDone(paymentIntentToken, pluginOption);
The pluginOption object
If you are using the default payment-container
, there is no need to specify a container
id in the pluginOption
object. If you are using a dynamic payment-container
, it is mandatory to provide a a container
id in the pluginOption
object or you will get errors. The theme
is optional in both cases, defaulting to classic
.
let pluginOption ={
theme : "classic", // classic, modern, minimal, vibrant
container: "DIV_ID" // replace with div id
}
Customizing AndDoneJS (Optional)
You can customize the appearance of the AndDoneJS widget to align with your brand using different themes in the pluginOption
object and by adjusting the CSS variables.
Using Themes
Start customizing plugins by picking a theme. The following themes are currently available:
- classic
- minimal
- modern
- vibrant
let pluginOption ={
theme : "classic", // classic, modern, minimal, vibrant
}
Using CSS variables
You can customize AndDoneJS by updating the CSS variables. Set the desired variable values to change the appearance of components visible in the AndDoneJS interface. You can inspect the resulting DOM using the DOM explorer in your browser.
You must include the CSS variable values in body *
.
<style>
body * {
--andDone-page-fontFamily: "Roboto";
--input-field-border-radius: 4px;
}
</style>
Commonly used variables:
Variable | Description |
--andDone-container-textColor |
The primary font color |
--andDone-page-fontFamily |
The primary font family |
--andDone-container-border-radius |
The border radius of the AndDoneJS container |
--andDone-label-fontWeight |
The font weight for the input label |
--input-field-fontSize |
The font size of the input fields |
--andDone-payButton-textColor |
The text color of the pay button |
--andDone-payButton-bgColor |
The background color of the pay button |
--tab-header-backgroundColor |
The background color of the tab bar |
--tab-header-active-tab-bgColor |
The background color of the active tab |
--tab-header-non-activeTab-BgColor |
The background color of the non-active tab |
--tab-header-non-activeTab-TextColor |
The text color of the non-active tab |
--tab-header-active-tab-text-color |
The text color of the active tab |
--input-error-borderColor |
The color used to display errors or destructive actions |
Process your first payment
The final step is to process your first test payment!
Start your server if it is not already running.
node server.js
Optionally, you can adjust the payment intent amount
or expiresIn
parameters in the constants.js
file.
Navigate to http://localhost:4200
in your browser. When the web page loads, the following actions occur:
- A payment intent request is submitted using the parameter values in constant.js.
- The payment intent is created and the
paymentToken
is returned to the server. - The server uses the
paymentToken
to initialize AndDoneJS. - The AndDoneJS widget is displayed on the web page.
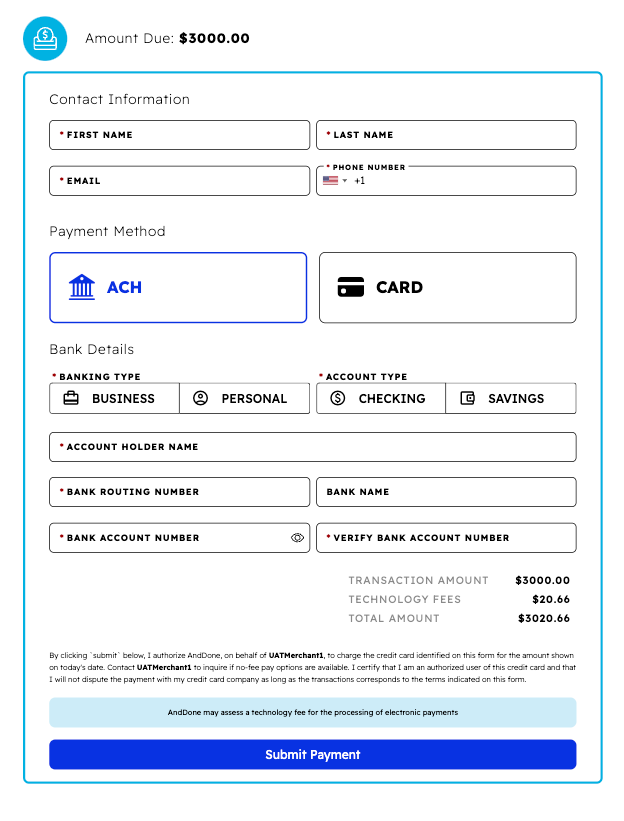
Enter 4000620000000007
as the test card number with an expiration date of 03/30
and 737
as the CVV Code, and click Submit Payment.
You have now submitted your first test payment using AndDoneJS, Congratulations!
Next Steps
Now that you have learned how to get AndDoneJS up and running in a test environment, you should be able to adapt these steps to fit into your production environment.